Recursion Function
Python Basics
Python Introduction
Python Installation
Overview of Jupyter IDE
Identifiers & Reserved Keywords
Python Variables
Python Numbers
Python Operators
Python Operators and Arithmetic Operators
Comparison and Logical Operators
Assignment and Bitwise Operators
Identity and Membership Operators
Python Flow Control
if else if else statement
While Loop Statement
Python For Loop
Break and Continue Statement
Python Data Types
Python Strings
Python Strings Methods
Python Lists
Python Tuples
Python Dictionary
Python Functions
Introduction to Python Functions
Function Arguments
Recursion Function
Lambda/Anonymous Function
Python - Modules
Python Files
Python - Files I/O
Python - Exceptions Handling
Python - Debugging
What is Recursion ?
Recursion is the process of defining something in terms of itself.
Eg: Two mirrors faced each other parallel. Any object in between them would be reflected recursively.
Python Recursive Function
We know that in Python, a function can call other functions. It is even possible for the function to call itself. These type of functions are called as recursive functions.
Flow Chart
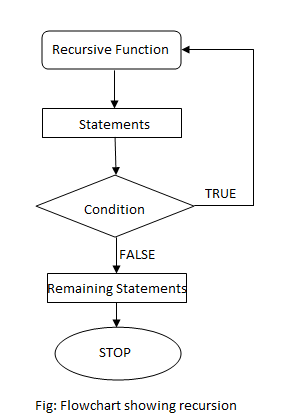
Example:
#Program to print factorial of a number using Recurion def factorial(num): """ This is a recursive function to find the factorial of a given number """ return 1 if num == 1 else (num * factorial(num-1)) # Getting the input from user during run time # Be default all the values will be changed to string num = input("Enter valid integer number: ") # Converting String to integer value num = int(num) print("The number entered is ", num) print("Factorial of {0} is {1} ".format(num, factorial(num))) Output: Enter valid integer number: 5 The number entered is 5 Factorial of 5 is 120
Advantages
- Recursive functions make the code look clean and elegant.
- A complex task can be broken down into simpler sub-problems using recursion.
- Sequence generation is easier with recursion than using some nested iteration.
Disadvantages
- Sometimes the logic behind recursion is hard to follow through.
- Recursive calls are expensive (inefficient) as they take up a lot of memory and time.
- Recursive functions are hard to debug.